利用するまでの流れ
まずは以下の様に Eclipse Plugin の Application Insight 機能をインストールする。

次に、以下の様に Azure の新管理ポータルで Application Insight を新規に作成した後、setting - プロパティ から Instrumentation Key を取得する。
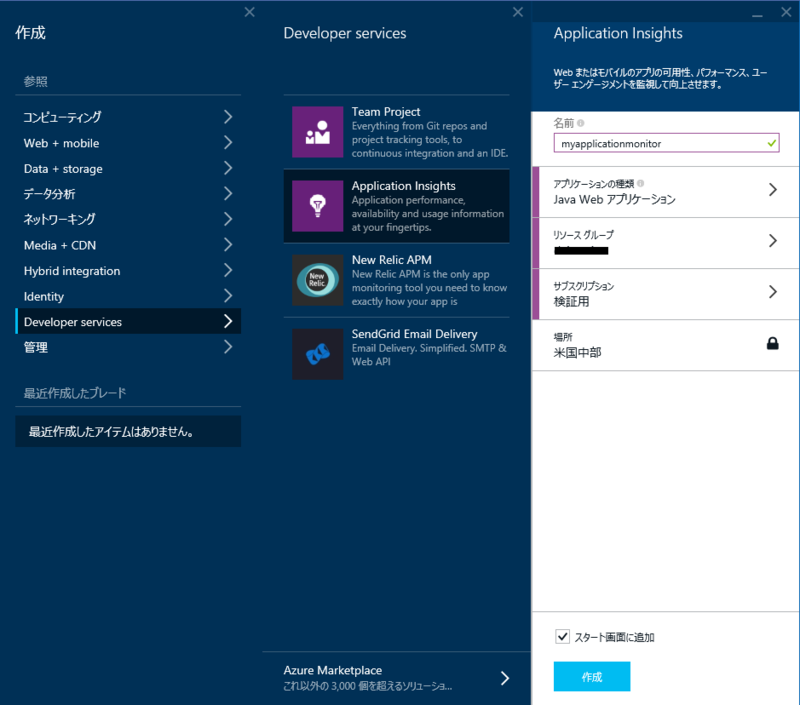
次は Eclipse 上から Dynamic Java Web アプリケーションを作成し、右クリックメニューから Configure Application Insight を選択して Application Insight有効化する。この際、Instrumentation Key を求められるので管理ポータルから取得しておくのを忘れないこと。

Application Insight 有効化すると、web.xml に以下の記載が追加されている。以下を見ると単なるサーブレットフィルタなので、Websites 等に配置せずとも動くことが推察できる。
xml version="1.0" encoding="UTF-8" standalone="no"
<web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlnsweb="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xmlnsxsi="http://www.w3.org/2001/XMLSchema-instance" id="WebApp_ID" version="3.0" xsischemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd">
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>ApplicationInsightsWebFilter</filter-name>
<filter-class>com.microsoft.applicationinsights.web.internal.WebRequestTrackingFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>ApplicationInsightsWebFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
更にソースフォルダに ApplicationInsights.xml が配置されており、以下の記載がされている。こちらで Application Insight を構成する情報が設定されていることが分かる(TelemetryInitializers タグにクラスを追加すると何かしてくれそうな気もする)。
xml version="1.0" encoding="utf-8" standalone="no"
<ApplicationInsights
xmlns="http://schemas.microsoft.com/ApplicationInsights/2013/Settings"
schemaVersion="2014-05-30">
<InstrumentationKey><自分のキーが書いてある>
</InstrumentationKey>
<ContextInitializers>
</ContextInitializers>
<TelemetryInitializers>
<Add
type="com.microsoft.applicationinsights.web.extensibility.initializers.WebOperationIdTelemetryInitializer" />
<Add
type="com.microsoft.applicationinsights.web.extensibility.initializers.WebOperationNameTelemetryInitializer" />
</TelemetryInitializers>
<TelemetryModules>
<Add
type="com.microsoft.applicationinsights.web.extensibility.modules.WebRequestTrackingTelemetryModule" />
<Add
type="com.microsoft.applicationinsights.web.extensibility.modules.WebSessionTrackingTelemetryModule" />
<Add
type="com.microsoft.applicationinsights.web.extensibility.modules.WebUserTrackingTelemetryModule" />
</TelemetryModules>
<Channel>
<DeveloperMode>false</DeveloperMode>
</Channel>
<DisableTelemetry>false</DisableTelemetry>
</ApplicationInsights>
この時点でも動作はするが Application Insight のクイックスタートの画面から以下の JavaScript が取得できるので、こちらを JSP 画面 or JSF 画面等の監視をしたいページに張り付ける。
<!--
ご使用のアプリケーションに関するエンド ユーザー利用状況分析を収集するには、
追跡するページごとに以下のスクリプトを挿入してください。
このコードを、</head> 終了タグの直前に、
他のスクリプトより前の位置に配置します。最初のデータは、数秒後に
自動的に表示されます。
-->
<script type="text/javascript">
var appInsights=window.appInsights||function(config){
function s(config){t[config]=function(){var i=arguments;t.queue.push(function(){t[config].apply(t,i)})}}var t={config:config},r=document,f=window,e="script",o=r.createElement(e),i,u;for(o.src=config.url||"//az416426.vo.msecnd.net/scripts/a/ai.0.js",r.getElementsByTagName(e)[0].parentNode.appendChild(o),t.cookie=r.cookie,t.queue=[],i=["Event","Exception","Metric","PageView","Trace"];i.length;)s("track"+i.pop());return config.disableExceptionTracking||(i="onerror",s("_"+i),u=f[i],f[i]=function(config,r,f,e,o){var s=u&&u(config,r,f,e,o);return s!==!0&&t["_"+i](config,r,f,e,o),s}),t
}({
instrumentationKey:"<自分のキーを利用する>"
});
window.appInsights=appInsights;
appInsights.trackPageView();
</script>
上記を利用してローカル等で Tomcat を起動して動作を確認すると、無事情報が取得できていることが分かる。
